When working with JavaScript for large projects, you may have noticed how difficult it becomes to maintain and scale code that has grown complex over time. Functions become hard to track, variables leak across scopes, and debugging takes longer. This is a common issue when code isn’t well-organized. This is where Object-Oriented Programming (OOP) steps in, offering a way to structure your code using objects and classes. With OOP principles such as inheritance, polymorphism, and encapsulation, JavaScript developers can build code that is easier to maintain, extend, and reuse.
JavaScript has evolved from a simple scripting language into a robust tool for building interactive web applications. Frameworks like React, Angular, and Node.js rely heavily on OOP principles to organize components, manage state, and build reusable modules. Mastering OOP concepts will make you more proficient in handling complex applications and keeping up with the industry’s demand for efficient development.
What is Object-Oriented Programming in JavaScript?
OOP is a paradigm that focuses on using objects—data structures that contain both properties (data) and methods (functions) to represent real-world entities. Unlike procedural programming, where you execute tasks step-by-step, OOP groups related data and behavior into objects, promoting better code structure.
In JavaScript, objects and classes form the backbone of OOP. Objects are collections of properties, and classes serve as blueprints for creating those objects.
JavaScript Classes and Objects: The Foundation of OOP
In ES6 (ECMAScript 2015), JavaScript introduced classes, making it easier to create objects. Here’s an example to illustrate:
class Car {
constructor(brand, model) {
this.brand = brand;
this.model = model;
}
describe() {
console.log(`This is a ${this.brand} ${this.model}.`);
}
}
const myCar = new Car("Toyota", "Corolla");
myCar.describe(); // Output: This is a Toyota Corolla.
Explanation:
class
: A class is a blueprint for creating objects.constructor
: This method initializes new objects with properties (in this case,brand
andmodel
).describe()
: This method defines behavior for the objects created from the class.
When you create a new instance using new Car()
, the constructor assigns initial values, and you can call methods like describe()
on the instance.
Encapsulation in JavaScript: Protecting Data
Encapsulation means restricting direct access to certain components of an object to protect data integrity. You can implement encapsulation in JavaScript using closures and private fields. Here’s an example using a closure:
function BankAccount(initialBalance) {
let balance = initialBalance;
this.getBalance = function() {
return balance;
};
this.deposit = function(amount) {
if (amount > 0) {
balance += amount;
}
};
}
const myAccount = new BankAccount(100);
console.log(myAccount.getBalance()); // Output: 100
myAccount.deposit(50);
console.log(myAccount.getBalance()); // Output: 150
Explanation:
- Here, the
balance
variable is private because it is only accessible throughgetBalance()
anddeposit()
methods. - Encapsulation ensures that the balance cannot be directly modified outside of the defined methods.
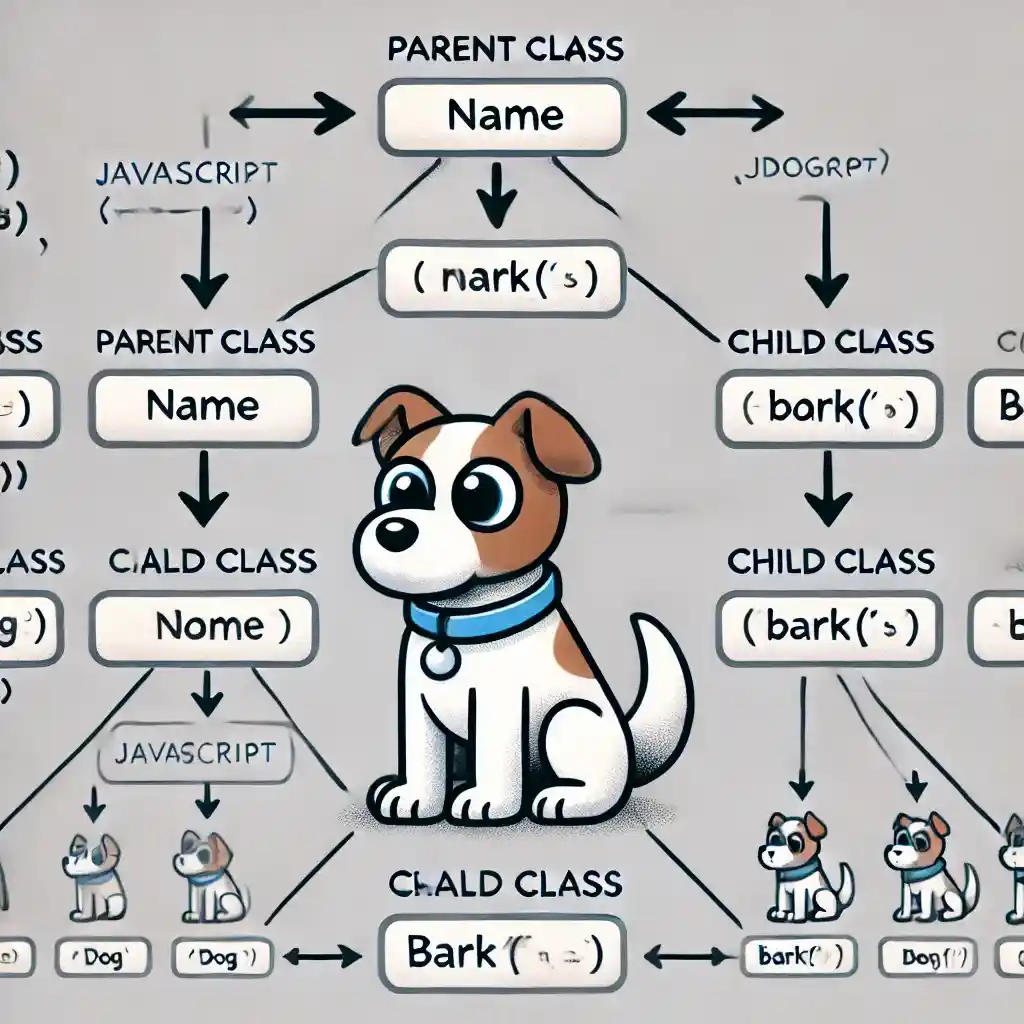
Inheritance in JavaScript: Code Reusability Through Extending Classes
JavaScript supports inheritance, allowing classes to inherit properties and methods from other classes using the extends
keyword.
class Animal {
constructor(name) {
this.name = name;
}
sound() {
console.log(`${this.name} makes a sound.`);
}
}
class Dog extends Animal {
sound() {
console.log(`${this.name} barks.`);
}
}
const myDog = new Dog("Rex");
myDog.sound(); // Output: Rex barks.
Explanation:
- The
Dog
class inherits fromAnimal
. - Even though both classes have a
sound()
method, the method inDog
overrides the one inAnimal
.
Polymorphism in JavaScript: Dynamic Behavior in Methods
Polymorphism allows objects to process behavior differently depending on their data type or class. This is achieved when a child class overrides a method from the parent class.
class Shape {
area() {
console.log("Calculating area...");
}
}
class Circle extends Shape {
constructor(radius) {
super();
this.radius = radius;
}
area() {
console.log(`Area of circle: ${Math.PI * this.radius ** 2}`);
}
}
const myCircle = new Circle(5);
myCircle.area(); // Output: Area of circle: 78.53981633974483
Explanation:
- Here, both
Shape
andCircle
have anarea()
method. - Calling
area()
on aCircle
instance invokes the overridden version, demonstrating polymorphism.
Abstraction in JavaScript: Simplifying Complex Logic
Abstraction is the concept of hiding unnecessary details and exposing only the essential parts. Although JavaScript does not have built-in abstract classes, you can simulate abstraction by designing interfaces with methods that subclasses must implement.
class Vehicle {
start() {
throw new Error("Method 'start()' must be implemented.");
}
}
class Bike extends Vehicle {
start() {
console.log("Bike is starting...");
}
}
const myBike = new Bike();
myBike.start(); // Output: Bike is starting...
Explanation:
- The
Vehicle
class provides a template, andBike
must implement thestart()
method. - This ensures abstraction, as each subclass must provide its specific implementation.
Constructors in JavaScript: Initializing Object States
Constructors are used to initialize an object’s properties when the object is created. They are called automatically when a new object is instantiated.
class Student {
constructor(name, grade) {
this.name = name;
this.grade = grade;
}
}
const student1 = new Student("John", "A");
console.log(student1.name); // Output: John
console.log(student1.grade); // Output: A
Explanation:
- The constructor assigns the initial state for each instance of the
Student
class.
Design Patterns in JavaScript: Best Practices for OOP
Design patterns are reusable solutions to common programming problems. Some popular OOP design patterns in JavaScript include:
- Singleton Pattern: Ensures only one instance of a class exists.
- Factory Pattern: Delegates object creation to specialized functions.
- Module Pattern: Encapsulates code into a single, reusable unit.
OOP vs Functional Programming in JavaScript: Which One to Use?
- OOP: Use when dealing with complex, stateful applications that require hierarchical structures (e.g., game development or web apps with multiple components).
- Functional Programming: Use for simpler, stateless functions (e.g., utility libraries or data transformations).
Both paradigms have their place, and often, a hybrid approach works best.
Conclusion
In this guide, we’ve explored the key concepts of Object-Oriented Programming in JavaScript, including encapsulation, inheritance, polymorphism, and abstraction. These principles allow developers to write cleaner, more maintainable code, making large projects easier to manage and extend. By incorporating OOP, you can improve both code quality and scalability in your projects.
Apply these OOP techniques to your next project to experience the benefits firsthand. As you become more familiar with design patterns and how to balance OOP vs functional programming, you’ll unlock new ways to build more efficient applications.